How to Create a Telegram Airdrop Bot: A Detailed Guide
Telegram is now essential for cryptocurrency projects. It helps with communication, builds communities, and manages airdrop campaigns. Airdrop bots on Telegram automate how tokens are shared. They also track user activity and ensure everything is clear. This guide shows you how to create a Telegram airdrop bot from the beginning. It includes the tools, the best ways to do it, and the technical details.
Part 1: Introduction to Telegram Airdrop Bots
What is a Telegram Airdrop Bot?
A Telegram airdrop bot makes it easy to give out free tokens to users on Telegram. It manages user sign-ups and tracks tasks like joining channels and following social media accounts. Tokens are then given out based on how much they participate.
Real-World Examples: Many DeFi tokens or NFT collections use airdrop bots to reward early users and create excitement.
Comparing Telegram Bots to Other Methods of Distribution
Method | Advantages | Disadvantages |
Telegram Bot | Automated, efficient, scalable, secure | Requires technical skills to develop |
Email Campaign | Wide reach, established infrastructure | Lower engagement rates, spam-prone |
Manual Distribution | Personalized, high control | Time-consuming, not scalable |
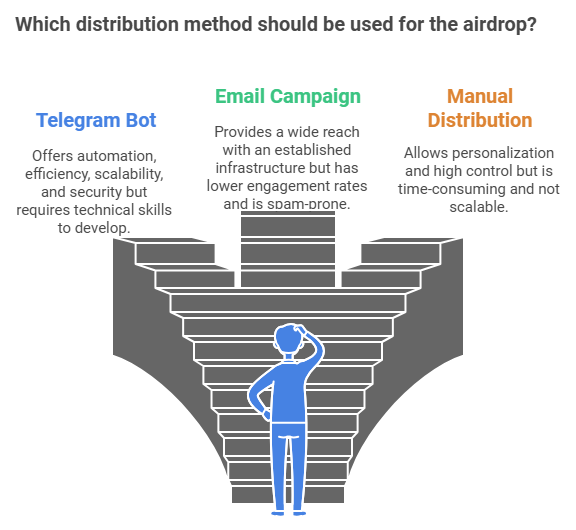
Part 2: Setting Up Your Telegram Bot
Prerequisites for Creating a Bot
Before diving in, ensure you have the following:
- Telegram Bot API: Use the official API to connect with Telegram.
- Hosting Service: A server for your bot to work all the time.
- Blockchain Wallet: To send tokens.
- Programming Knowledge: Basic skills in Python or JavaScript (Node.js).
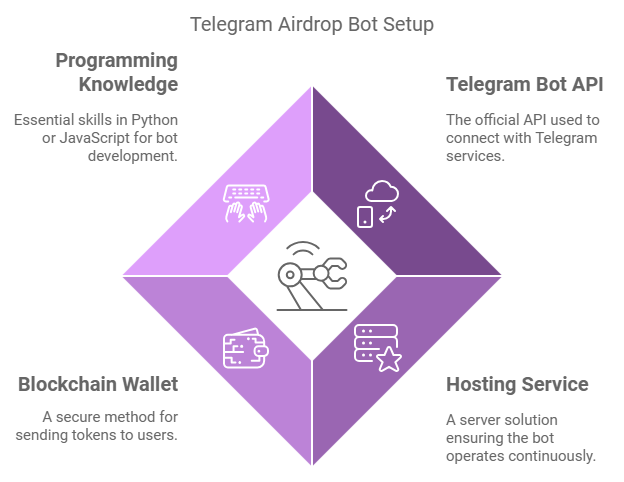
Hosting Platform Comparison
Platform | Advantages | Disadvantages |
Heroku | Easy setup, free tier, good for small projects | With limited resources on the free tier, scaling can be costly |
AWS | Scalable, powerful | With limited resources on free tier, scaling can be costly |
DigitalOcean | Affordable, simple interface | Fewer features compared to AWS |
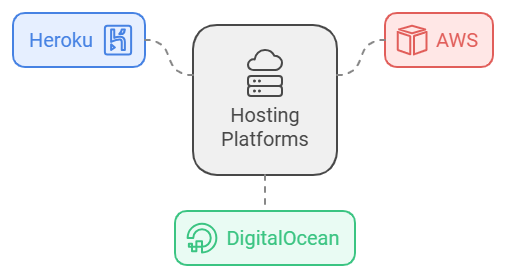
Hosting Platform Advantages & Disadvantages
Heroku
Advantages:
- Easy Setup: Heroku is easy to use. It has a friendly interface. You can deploy your apps with little setup, which is good if you do not want to waste time on server management. It is a great choice for beginners who are new to development. It is also good for people who do not want to handle the tricky parts of infrastructure.
- Free Tier: Heroku has a free tier. This is great for small projects, prototypes, or development work. With this tier, you can deploy your bot without paying anything upfront. The free tier is good for testing your bot and trying things out before you grow to a bigger audience.
- Good for Small Projects: Heroku is great for small to medium bots with light traffic. Its managed environment has everything you need without having to deal with your own servers. It takes care of things like scaling and load balancing for you, so you do not need to set those up yourself.
Disadvantages:
- Limited Resources on Free Tier: – The free tier looks good, but it has big limits. You will get less computing power, limited memory, and a cap on add-ons (extra services like databases or monitoring tools).
- Also, if your app is inactive for 30 minutes, it goes to sleep. This can cause delays when you try to start the bot again after a break.
- Scaling Can Be Costly: – As your bot gets bigger and needs to support more users, you may need to switch to a paid plan. Heroku can get pricey as you grow, especially when you need more resources like more dynos (virtual servers) and add-ons like databases.
- The costs can add up fast, making it harder for large projects or when you need high availability.
AWS (Amazon Web Services)
Advantages:
- Scalable and Powerful: – AWS is a strong cloud platform that can grow with you. You can easily add more resources as more people use your Telegram bot. This is true for both small projects and larger business applications.
- AWS has many services like EC2 (Elastic Compute Cloud), RDS (Relational Database Service), and S3 (Simple Storage Service). These help you have full control over your system.
- Flexibility and Customization: – AWS gives you good options to customize how you host your bot. You can pick the right resources for what you need, like CPU, RAM, and storage.
- It also offers great control over networking, security, and balancing tasks, so you can adjust every part of your system to fit what you want.
- Global Reach: – With AWS, you can set up your bot in many parts of the world. This way, you can better serve users from different places, and it helps keep wait times low and responses quick.
Disadvantages:
- Complex Setup: AWS is powerful, but it’s also hard to learn. Setting up your systems, like configuring EC2 instances, security groups, and databases, can be tricky and take a lot of time. For beginners or anyone with less experience, the platform might feel too much without good knowledge of cloud computing.
- Can Become Expensive: AWS has a pay-as-you-go plan, which can lead to surprise costs if you’re not careful. The price depends on the services you use, so if you don’t keep an eye on it, your bill can grow as your usage goes up. To manage costs well, you need careful planning and knowledge of AWS’s billing system, especially for services like EC2 or S3.
DigitalOcean
Advantages:
- Affordable: DigitalOcean is known for its low pricing. Its pricing is easy to understand, which helps you manage costs.
- You can start with a small plan (as low as $5 per month) and grow as you need. This makes it a good choice for developers who want to keep costs down while getting the resources they need.
- Simple Interface: DigitalOcean has a clear and easy dashboard. You don’t need to be an expert to deploy and manage your bot there.
- This platform is good for developers who like easy use and simple management instead of many features.
- Great for Small to Medium Projects: DigitalOcean’s droplets (private servers) work well for small to medium apps. If you have a bot with average traffic, DigitalOcean offers a good mix of performance and cost.
Disadvantages:
- Fewer Features Compared to AWS:- DigitalOcean is simpler. However, it does not offer as many advanced services as AWS. For instance, it has fewer managed databases and limited networking options. If your bot grows a lot or if you need special services, like machine learning or better security, you might feel restricted by what DigitalOcean offers.
- Less Flexibility:- DigitalOcean is easy to use. But it allows for less custom change than AWS. You might feel limited if you need to change the server setup for special needs, such as better network features or security arrangements.
Part 3: Building the Bot’s Logic
Step 1: Creating Your Bot with BotFather
- Open Telegram and look for “BotFather.”
- Start a chat and type.
/newbot
. - Follow the steps to name your bot and choose a username. Save the special API token.
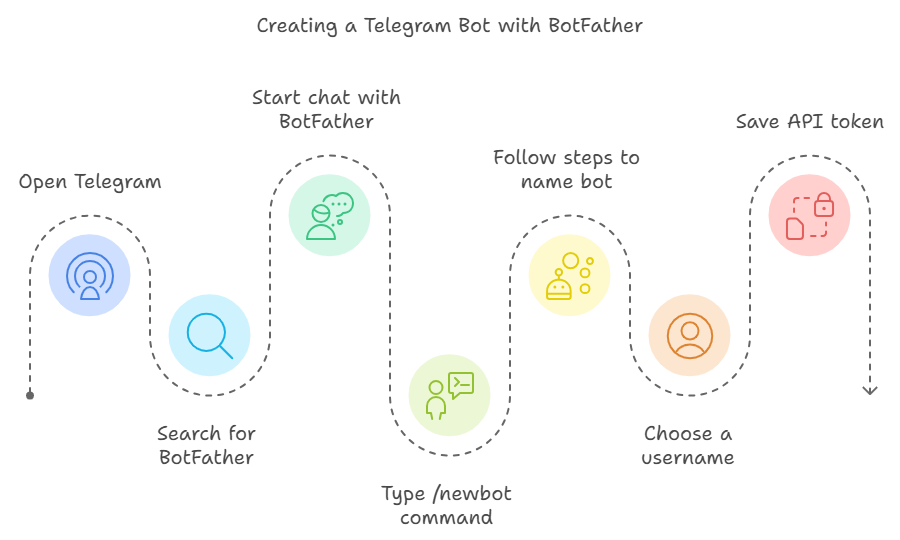
Step 2: Setting Up Your Development Environment
- Python Installation: You need to install
<strong>python-telegram-bot</strong>
and **<strong>pymongo</strong>
to manage your database. - Node.js Installation: Use
npm
oryarn
to get the Telegram Bot API library and a database driver. - Database Setup: MongoDB is a good choice for beginners.
Step 3: Writing the Core Bot Code
from telegram import Update
from telegram.ext import Updater, CommandHandler, CallbackContext, MessageHandler, Filters
import logging
# Enable logging for debugging
logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
level=logging.INFO)
logger = logging.getLogger(__name__)
# Replace with your bot token
TOKEN = 'YOUR_BOT_TOKEN'
def start(update: Update, context: CallbackContext) -> None:
update.message.reply_text('Welcome to the Telegram Airdrop Bot! Use /airdrop to begin.')
def airdrop(update: Update, context: CallbackContext) -> None:
try:
user = update.message.from_user
if is_user_registered(user.id): # Implement user registration check
update.message.reply_text("You've already registered!")
return
register_user(user.id, user.username) # Implement user registration
update.message.reply_text(f'{user.username}, you have started the airdrop! Please provide your wallet address.')
context.user_data['next_stage'] = 'waiting_for_wallet_address'
except Exception as e:
logger.error(f"Error in airdrop command: {e}")
update.message.reply_text("An error occurred. Please try again later.")
def handle_text_message(update: Update, context: CallbackContext) -> None:
if 'next_stage' in context.user_data and context.user_data['next_stage'] == 'waiting_for_wallet_address':
wallet_address = update.message.text
if is_valid_wallet_address(wallet_address): # Implement wallet validation
store_wallet_address(update.message.from_user.id, wallet_address) # Implement wallet storage
update.message.reply_text("Wallet address received. Please complete the following tasks.")
del context.user_data['next_stage']
else:
update.message.reply_text("Invalid wallet address. Please provide a valid address.")
Step 4: Implementing Airdrop Functionality
- Collect User Information: Check wallet addresses using regex or tools like
web3.py
. - Track Task Completion: Keep track of task completion status in your database for real-time updates.
- Check Task Completion: Make sure users finish all tasks before they get tokens.
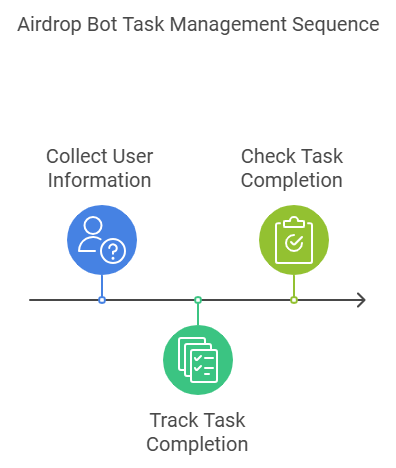
Step 5: Token Distribution
- Blockchain Integration: Use Web3.py or ethers.js to work with your smart contract and send tokens.
- Gas Estimation: Always estimate gas fees to make sure transactions go through.
Part 4: Security and Best Practices
Anti-Spam Measures
- Use CAPTCHAs to stop bots from hurting your site.
- Use rate limiting to keep spammy actions away.
Error Handling
- Record errors for fixing problems and use complete error handling to keep the bot working.
Security Best Practices
- Encrypt important data like wallet addresses using tools like cryptography.
- Regularly do tests and checks for security.
Part 5: Hosting, Deployment, and Maintenance
- Scalable Hosting: Pick a hosting service that grows with your needs (for example, AWS or Heroku).
- Monitoring Tools: Utilize tools to watch performance and uptime, especially as you expand your bot.
Part 6: Engagement and Gamification
Gamification
- Use leaderboards, quizzes, and reward tiers to increase excitement and participation.
Referral Systems
- Ask users to invite others using special invitation links. Give rewards to both the person who is invited and the person who is invited.
Personalized Messaging
- Change messages to fit how users are doing and how much they are involved.
Part 7: FAQs
Use CAPTCHAs, track IP addresses, and watch user behavior.
Issues with checking inputs, weak data storage, and not enough error handling.
Choose upgradable hosting, optimize database searches, and use caching plans.
Part 8: Case Studies and Advanced Techniques
Case Study 1: Successful Airdrop Bot
- Look at a good campaign. Focus on parts like task tracking, fun activities, and ways to keep users interested.
Case Study 2: Failed Airdrop Campaign
- Learn from a failed effort and find lessons to boost safety, check tasks, and engage users better.
Advanced Techniques
- Look into multi-platform integration (like Discord or Twitter) and set up reward systems that change based on how active users are or how fast they finish tasks.
Conclusion
Building a Telegram airdrop bot is a great way to connect with your crypto community while sharing tokens. By using this guide and following good practices, you can also make your airdrop campaigns more fun and exciting. This guide gives you everything you need to do well, whether you are just starting or want to grow.
Hello there! I know this is kind of off topic but I was wondering if you knew
where I could get a captcha plugin for my comment form?
I’m using the same blog platform as yours and I’m having problems finding one?
Thanks a lot!